Getting Started with Authentication in React Native
11/11/2024
42
Authentication is a critical part of most mobile applications. It helps verify user identity and control access to data and features. There are several libraries that make it easier to set up authentication in React Native. This guide will walk you through the basics of authentication, using the popular libraries react-native-app-auth
and Auth0.
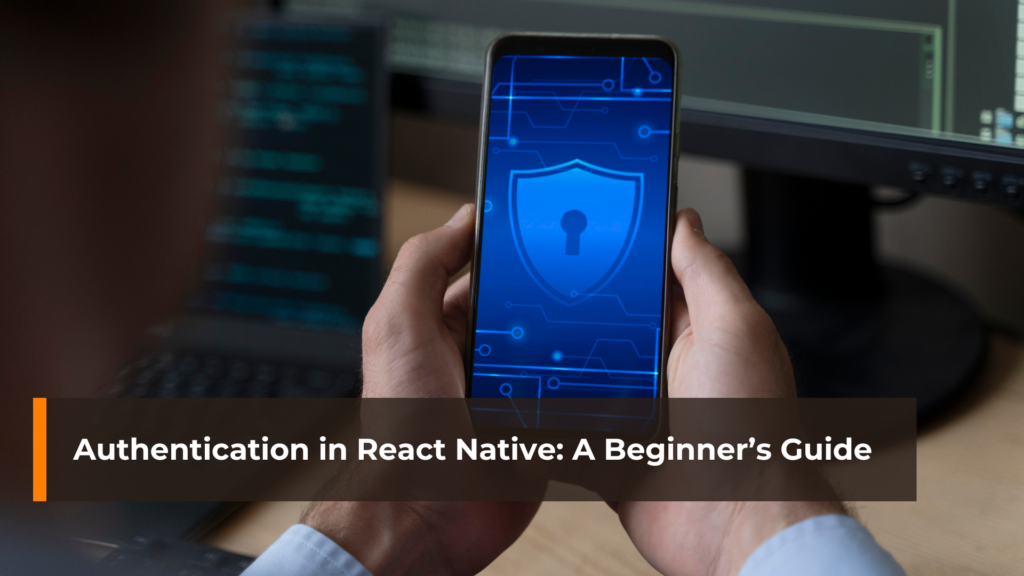
Why Use an Authentication Library?
Using an authentication library simplifies the process of managing user credentials, tokens, and permissions. It also adds security, as these libraries follow the latest standards and best practices. Here, we’ll explore react-native-app-auth
for OAuth 2.0 authentication and Auth0 for a more comprehensive identity management solution.
Setting Up Authentication with react-native-app-auth
react-native-app-auth
is a library that supports OAuth 2.0 and OpenID Connect. It’s suitable for apps that need to connect with Google, Facebook, or other providers that support OAuth 2.0.
Installation
Start by installing the library with:
npm install react-native-app-auth
If you’re using Expo, you’ll need to use expo-auth-session
instead, as react-native-app-auth
is not compatible with Expo.
Basic Setup
To set up react-native-app-auth
, configure it with the provider’s details (e.g., Google):
import { authorize } from 'react-native-app-auth'; const config = { issuer: 'https://accounts.google.com', // Google as OAuth provider clientId: 'YOUR_GOOGLE_CLIENT_ID', redirectUrl: 'com.yourapp:/oauthredirect', scopes: ['openid', 'profile', 'email'], };
In this configuration:
issuer
is the URL of the OAuth provider.clientId
is the ID you receive from the provider.redirectUrl
is the URL your app redirects to after authentication.scopes
defines what data you’re requesting (e.g., user profile and email).
Implementing the Login Function
With the configuration done, create a function to handle login:
const login = async () => { try { const authState = await authorize(config); console.log('Logged in successfully', authState); // Use authState.accessToken for secure requests } catch (error) { console.error('Failed to log in', error); } };
Here:
authorize(config)
triggers the authentication flow.- If successful,
authState
contains the access token, ID token, and expiration date. - Use the
accessToken
to make requests to the API on behalf of the user.
Logging Out
To log users out, clear their tokens:
const logout = async () => { try { await authorize.revoke(config, { tokenToRevoke: authState.accessToken }); console.log('Logged out'); } catch (error) { console.error('Failed to log out', error); } };
This will remove the access token and effectively log out the user.
Setting Up Authentication in React Native with Auth0
Auth0 is a widely used identity provider that offers a more comprehensive authentication setup. It supports multiple login methods, such as social login, username/password, and enterprise authentication.
Installation
Install the Auth0 SDK for React Native:
npm install react-native-auth0
Basic Setup
Initialize the Auth0 client by providing your domain and client ID:
import Auth0 from 'react-native-auth0'; const auth0 = new Auth0({ domain: 'YOUR_AUTH0_DOMAIN', clientId: 'YOUR_CLIENT_ID', });
Implementing the Login Function
Use Auth0’s web authentication method to start the login flow:
const login = async () => { try { const credentials = await auth0.webAuth.authorize({ scope: 'openid profile email', audience: 'https://YOUR_AUTH0_DOMAIN/userinfo', }); console.log('Logged in successfully', credentials); // Store credentials.accessToken for API requests } catch (error) { console.error('Failed to log in', error); } };
Here:
scope
andaudience
define the permissions and data you request.credentials.accessToken
will be used for secure API requests.
Logging Out
To log out with Auth0:
const logout = async () => { try { await auth0.webAuth.clearSession(); console.log('Logged out'); } catch (error) { console.error('Failed to log out', error); } };
Storing Tokens Securely
Tokens are sensitive data and should be stored securely. Use libraries like react-native-keychain
or SecureStore
in Expo to securely store tokens:
import * as Keychain from 'react-native-keychain'; const storeToken = async (token) => { await Keychain.setGenericPassword('user', token); }; const getToken = async () => { const credentials = await Keychain.getGenericPassword(); return credentials ? credentials.password : null; };
Conclusion
This guide covered setting up basic authentication in React Native with react-native-app-auth
and Auth0. These libraries streamline the process of handling secure login and token management. After implementing, remember to handle token storage securely to protect user data.
Streamline Authentication in React Native with SupremeTech’s Offshore Development Expertise
Setting up authentication in a React Native app can be complex, but with the right libraries, it’s achievable and secure. Whether using react-native-app-auth
for OAuth 2.0 or Auth0 for comprehensive identity management, these tools help handle user authentication smoothly and securely.
For businesses aiming to scale and streamline mobile app development, SupremeTech offers skilled offshore development services, including React Native expertise. Our teams are experienced in building secure, high-performance applications that meet industry standards. If you’re looking to enhance your mobile development capabilities with a trusted partner, explore how SupremeTech can support your growth.
Related Blog